The core function of DevOps is to facilitate and automate the process of delivering code that developers write into production. Where CI/CD is involved, that means that code is compiled, tested and packaged, as applicable, whenever code is committed to a repository. A DevSecOps approach integrates security into the DevOps process through such steps as static application code analysis, dependency checking and dynamic application testing. A more recent concept, GitOps aims to use Git as the source for declarative and automated management of infrastructure and application deployments, where the live state of the system matches the desired state specified in the Git repository.
It can be daunting to be tasked with setting up an automated, secure, Git-based workflow to deploy services to a Kubernetes cluster. This article and the ones that follow aim to take the apprehension out of this process by providing step-by-step guidance. By the end of this series, you will have a solid understanding of how to set up an efficient, secure, and automated pipeline that seamlessly deploys code to a Kubernetes cluster, empowering you to confidently tackle complex deployments with ease.
The article is titled “From zero to 99” and not 100 because this is after all a demo app, and real-world situations will require additional work. However, the concepts here should get you nearly all the way there. By approaching the task like any large software project and breaking it down into subtasks that give us quick wins, you will feel like you are making progress and you can take the time to explore variations to what you have already built. While the specifics of your situation might differ from this example, it shouldn’t matter, because the principles will remain the same. For example, this guide uses GitHub Actions for the CI workflow, but you can achieve the same results using Bitbucket Pipelines or Jenkins or Circle CI.
Prerequisites
The purpose of this guide is to set up a workflow that deploys code to a Kubernetes cluster if all the tests pass and there are no security issues. The workflow relies on many tools and platforms as prerequisites and this guide expects them to already be installed and set up. Going into the details of how to set up the prerequisites is beyond the scope here.
- Python
- Docker
- Kubernetes cluster
- kubectl, helm
- Github account
- Github personal access token for ghcr.io authentication
Subtasks
We will approach the task of setting up the workflow by dividing it up into subtasks. Each of these will be a small win that will give us a sense of progress and accomplishment.
- Create an application (Hello World Flask app)
- Containerize the app (Docker)
- Deploy to a Kubernetes cluster (Minikube)
- Set up the CI workflow (Github Actions)
- Set up CD with GitOps (ArgoCD)
Create a Python application
Let’s get started with the first subtask. For this exercise, we will use a simple Hello World web app written in Python and using the Flask library. In a real scenario, this can be expanded to a full app that does much more than return some text.
from flask import Flask
app = Flask(__name__)
@app.route("/")
def hello():
return "<p>AllĂ´, le monde!</p>"
if __name__ == "__main__":
# Only for debugging while developing
app.run(host="0.0.0.0", debug=True, port=8080)
Code language: Python (python)
Save the file as main.py. We also need to install the flask library. If you do not already have a virtual environment, run the following commands:
virtualenv -p python3 venv
source ./venv/bin/activate
pip install flask
Now we can test out the app. Run
python main.py
Code language: plaintext (plaintext)
You should see something similar to the following:
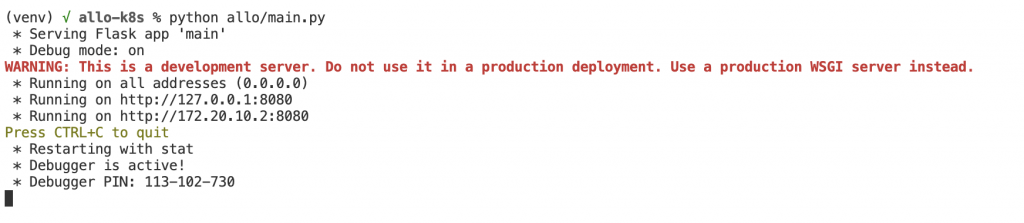
Open a browser window and navigate to http://localhost:8080 or click on the link. You should see something like the following:
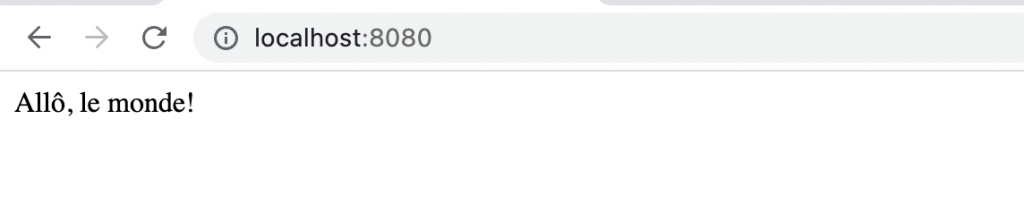
If you see your hello message, congratulations, you have a working app! It is not a CI/CD workflow yet, but chalk up small win number one!
In the next article, we will see how to containerize the app, which will then allow us to deploy it almost anywhere.